Author: Dhruv Shrivastava
The Dice is a simple cube shaped like object used in various board games such as snake- ladder, Ludo etc. It is a simple cube which generates a random number when rolled by a user. A Dice rolling simulator is nothing but a computer model that can be created by a software program and it functions same as a normal dice in which user rolls a dice and a random number gets shown on the screen. There are many ways a dice simulator can be implemented. In this paper, we will implement a GUI Application of dice simulator using Tkinter Framework in Python.
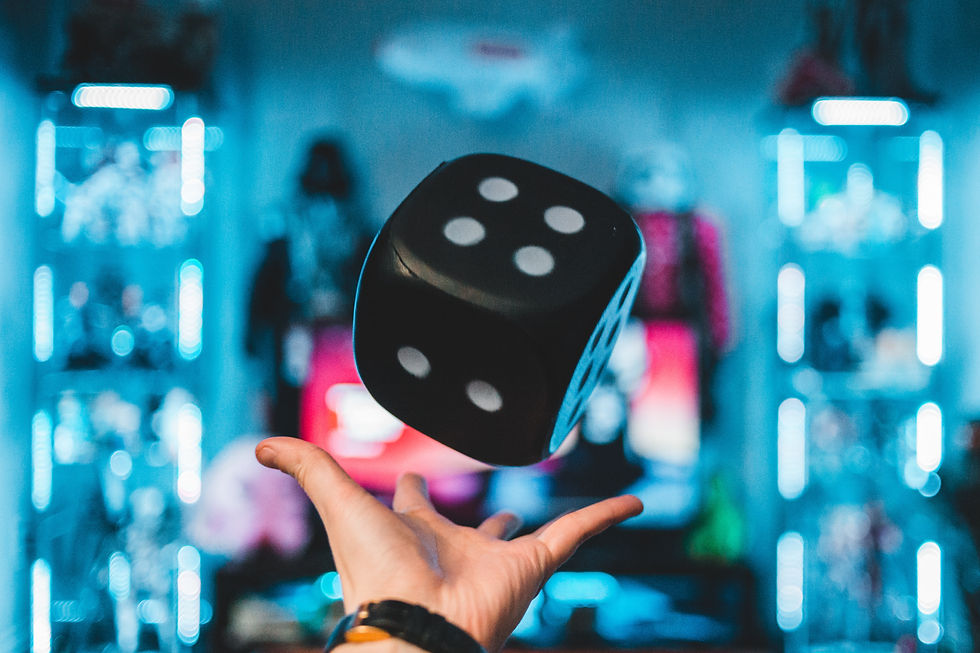
What is Tkinter?
Python has various frameworks/packages which can be used for making GUI applications i.e., the Graphical User Interface. Tkinter is the standard GUI package for Python. It is the most common, fast, and easy way to create a GUI (Graphical User Interface) application. It provides a powerful object-oriented interface to the Tk GUI toolkit. We can develop an application and can use that application on any platform, which reduces the need for additional requirements required to use an application on any OS like Windows, Mac, or Linux.
What is GUI (Graphical User Interface)?
The Graphical User Interface (GUI) is a form of user interface which allows a user to interact with electronic devices with the help of graphical icons and visual components. It displays objects that conveys information, and displays actions performed by the user.
Dice Rolling Simulator implementation
Everyone knows what a dice. It’s a simple cube like structure which displays random number from 1 to 6 when rolled. So, The dice simulator is nothing but a computer model that can roll a dice for us.
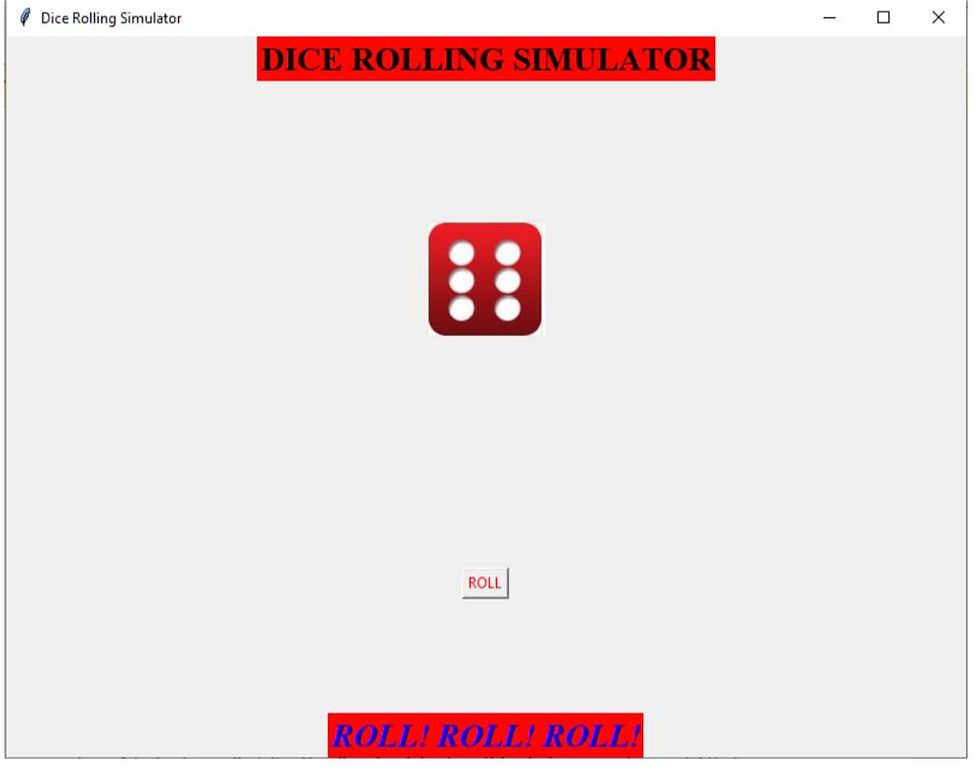
FIG:1
How to Implement the Dice rolling Simulator?
Step-1: Importing the required modules which will be required
The following modules to be imported are:
Tkinter: Used for accessing Tkinter and make GUI applications
Image, Imagetk: Imported from Python Imaging Library. We use it to perform operations with images in our UI
Random: It is imported to generate random numbers.
Code:

FIG:2
Step-2:
Creating a top-level Widget that will be the main window for our application.
We will build the main window of our application, where the buttons, labels, and images will reside. We will also create a title with the title() function.

FIG:3
The output of the above code will be: the above code will generate a blank window of size defined in geometry(‘800x600’) with the title Dice Rolling Simulator’.
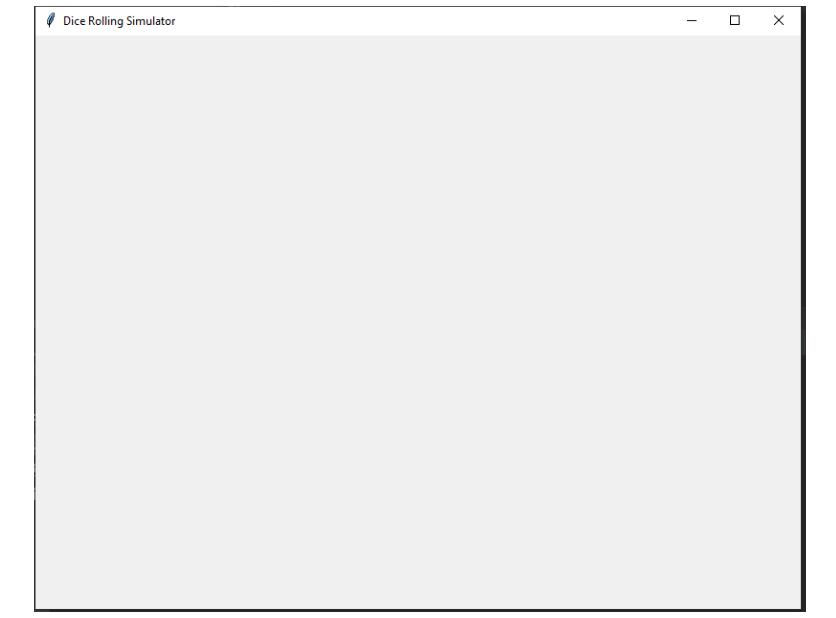
FIG:4
Step3:
In upcoming steps, we will create different labels and add images to our main window which will be displayed on our application. Also, we will create buttons in our application that will roll the dice. First, we will create different labels:
Code:
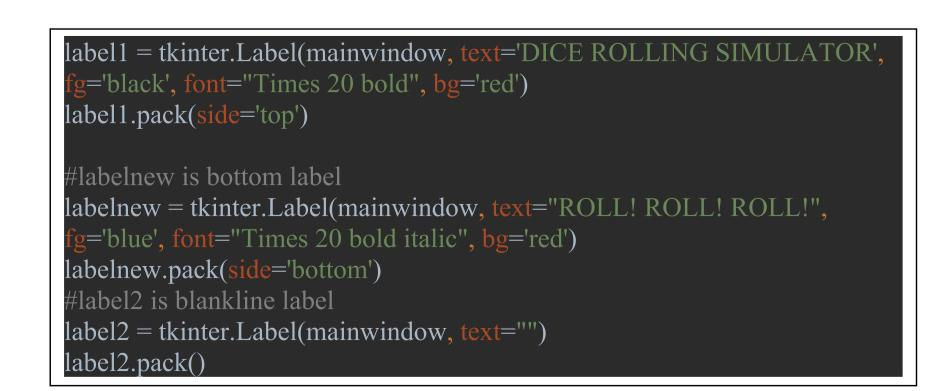
FIG:5
Here, we have used pack() to arrange our widgets in row and column form in our main window. The Heading label is label1 which will give heading in our application and ‘BlankLine’ label is to skip a line. ‘Bottom’ label is ‘labelnew’ which will appear in bottom of our application.
Mainwindow: it is the name we refer to the mainwindow of the application. You can name it with anything.
Text: it is the text to be displayed in the labels we created.
bg: background colour of the label text.
fg: the colour of the font that we used.
Font: in this we define the font of label text.
.pack(): it is used to pack the widget onto the mainwindow
Output:
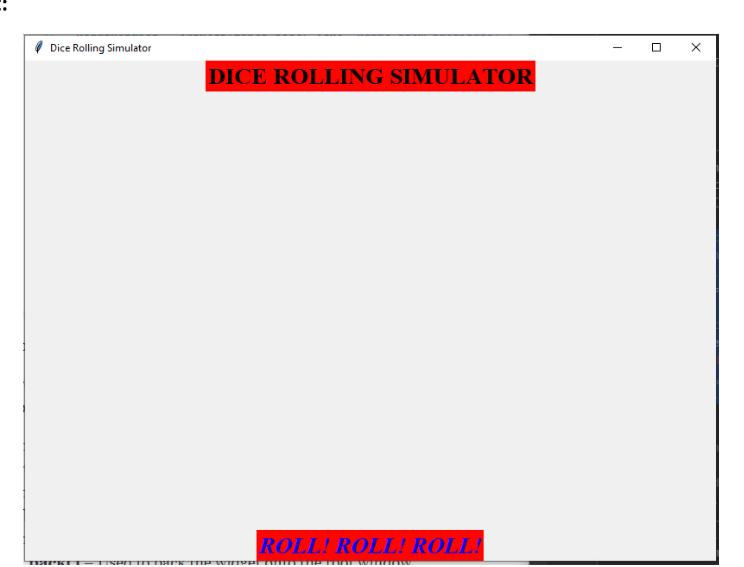
FIG:6
Step 4:
Creating a list of images to be randomly displayed
Code:
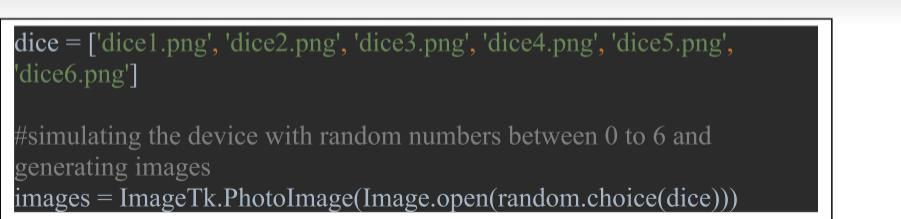
FIG:7
‘Dice’ is the list of images that are in the same folder, and are chosen randomly according to the random numbers generated by ‘random. choice’. ‘images’ is created to store an image of dice which is generated randomly.
Step 5:
Creating a label for the Image, adding a button, and assigning a function to it.
Code:
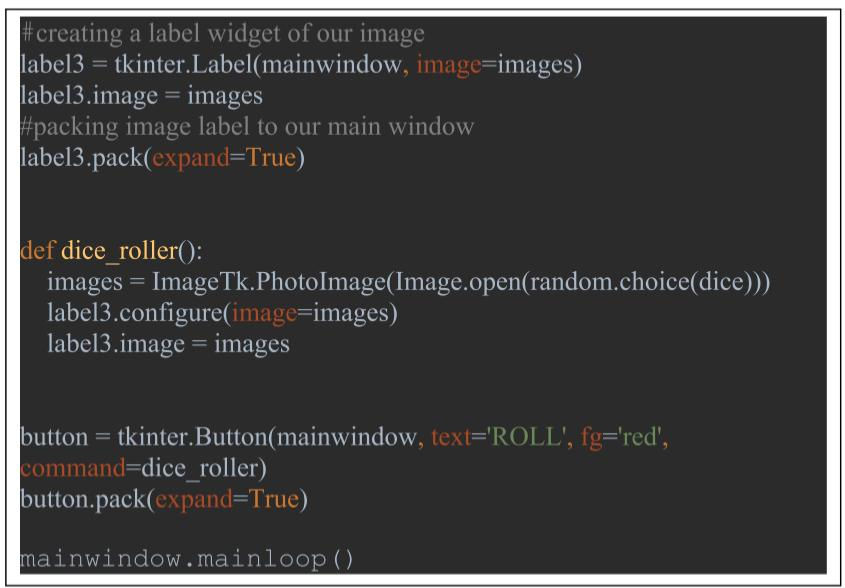
FIG:8
Final output:
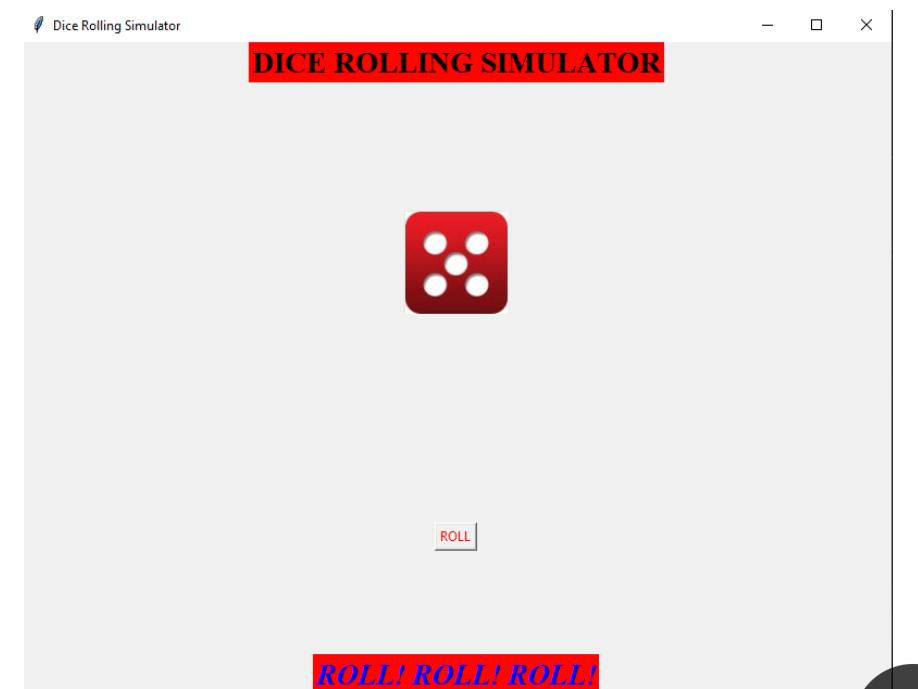
FIG:9
GitHub Link:
https://github.com/dhruv20032000/Dice-Rolling-Simulation
References:
https://en.wikipedia.org/wiki/Tkinter
https://www.tutorialspoint.com/python/python_gui_programming.htm
https://www.w3schools.com/python/ref_random_choice.asp
https://data-flair.training/blogs/dice-rolling-simulator-python/
https://pillow.readthedocs.io/en/stable/reference/ImageTk.html
http://clipart-library.com/dice-faces.html
Comments